# FastAPI - The Complete Course 2025 (Beginner + Advanced) 🚀
Welcome to the **FastAPI - The Complete Course 2025 (Beginner + Advanced)** repository! This space is dedicated to the code developed throughout the course offered on Udemy. Whether you're just starting or looking to refine your skills, this repository has you covered.
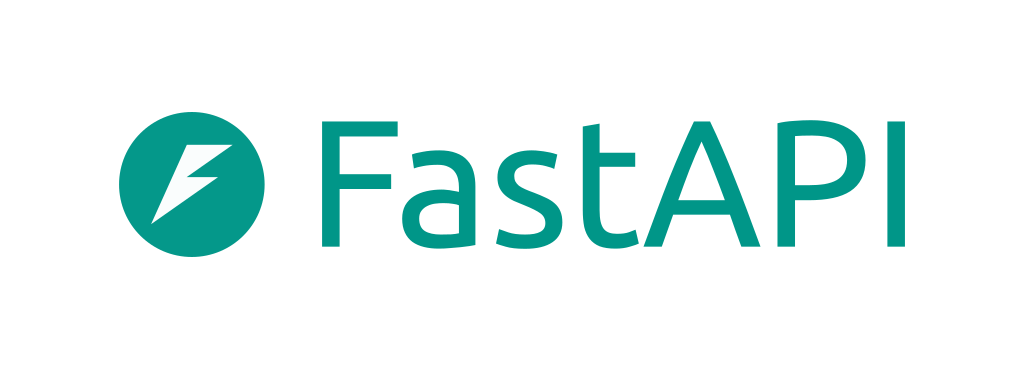
## Table of Contents
- [Course Overview](#course-overview)
- [Getting Started](#getting-started)
- [Installation](#installation)
- [Usage](#usage)
- [Folder Structure](#folder-structure)
- [Code Examples](#code-examples)
- [Contributing](#contributing)
- [License](#license)
- [Links and Resources](#links-and-resources)
- [Contact](#contact)
## Course Overview
FastAPI is a modern, fast (high-performance) web framework for building APIs with Python 3.6+. It is based on standard Python type hints. The course aims to teach you how to create APIs efficiently and effectively. By the end of this course, you'll have the knowledge to build and deploy your applications.
### What You Will Learn
- How to set up FastAPI and create your first API.
- Work with path parameters and query parameters.
- Understand request and response models using Pydantic.
- Implement authentication and authorization.
- Handle database operations with SQLAlchemy.
- Create and manage Docker containers for your applications.
## Getting Started
To get started, clone this repository to your local machine:
```bash
git clone https://github.com/ashok14k/FastAPI-The-Complete-Course-2025-Beginner-Advanced-Udemy.git
Navigate to the directory:
cd FastAPI-The-Complete-Course-2025-Beginner-Advanced-Udemy
Ensure you have Python 3.6+ installed. You can check your Python version with:
python --version
Next, install the required packages. It is best to use a virtual environment:
python -m venv venv
source venv/bin/activate # On Windows use `venv\Scripts\activate`
pip install -r requirements.txt
Run the FastAPI application with the following command:
uvicorn main:app --reload
Visit http://127.0.0.1:8000/docs
to explore the interactive API documentation generated by FastAPI.
The repository follows a structured approach to make navigation easier. Below is an overview of the main folders and files:
.
├── app/
│ ├── main.py
│ ├── models/
│ ├── routers/
│ ├── schemas/
│ └── utils/
├── requirements.txt
└── README.md
app/main.py
: The main application file where the FastAPI app instance is created.app/models/
: Contains database models.app/routers/
: Houses the API route definitions.app/schemas/
: Holds Pydantic models for request and response validation.app/utils/
: Any utility functions.
Here are some snippets to illustrate common tasks:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"Hello": "World"}
from pydantic import BaseModel
class User(BaseModel):
id: int
name: str
email: str
from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
@app.post("/token")
async def login(form: OAuth2PasswordRequestForm = Depends()):
# Perform authentication
pass
We welcome contributions! To get started:
- Fork the repository.
- Create a new branch.
- Make your changes.
- Submit a pull request.
Please ensure your code follows the existing style and includes tests.
This project is licensed under the MIT License. See the LICENSE file for details.
For more information, visit the following:
You can download the latest releases and updates from our Releases page.
For questions or feedback, feel free to reach out through GitHub.
Happy coding! 💻