You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
In this project we adapt the model from [Choi et al.](https://github.com/keunwoochoi/music-auto_tagging-keras) to train a custom music genre classification system with our own genres and data. The model takes as an input the spectogram of music frames and analyzes the image using a Convolutional Neural Network (CNN) plus a Recurrent Neural Network (RNN). The output of the system is a vector of predicted genres for the song.
6
+
7
+
We fine-tuned their model with a small dataset (30 songs per genre) and test it on the GTZAN dataset providing a final accuracy of 80%.
In this repository we provide the scripts to fine-tune the pre-trained model and a quick music genre prediction algorithm using our own weights.
18
+
19
+
Currently the genres supported are the [GTZAN dataset](http://marsyasweb.appspot.com/download/data_sets/) tags:
20
+
21
+
- Blues
22
+
- Classical
23
+
- Country
24
+
- Disco
25
+
- HipHop
26
+
- Jazz
27
+
- Metal
28
+
- Pop
29
+
- Reggae
30
+
- Rock
31
+
32
+
### Prerequisites
33
+
34
+
We have used Keras running over Theano to perform the experiments. Was done previous to Keras 2.0, not sure if it will work with the new version. It should work on CPU and GPU.
35
+
- Have [pip](https://pip.pypa.io/en/stable/installing/)
Python packages necessary specified in *requirements.txt* run:
39
+
```
40
+
# Create environment
41
+
virtualenv env_song
42
+
# Activate environment
43
+
source env_song/bin/activate
44
+
# Install dependencies
45
+
pip install -r requirements.txt
46
+
47
+
```
48
+
49
+
### Example Code
50
+
51
+
Fill the folder music with songs. Fill the example list with the song names.
52
+
```
53
+
python quick_test.py
54
+
55
+
```
56
+
57
+
## Results
58
+
59
+
### Sea of Dreams - Obenhofer
60
+
[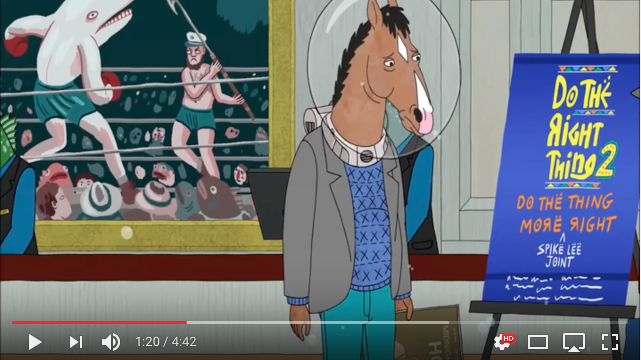](https://www.youtube.com/watch?v=mIDWsTwstgs)
[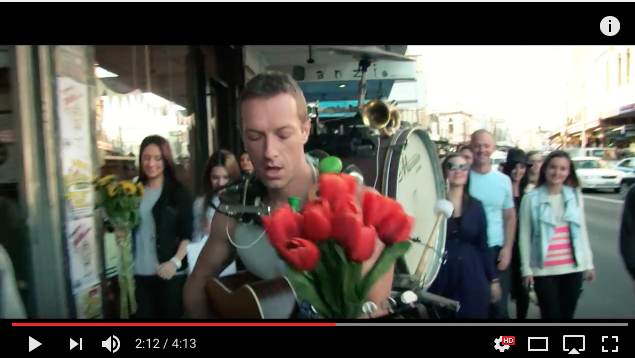](https://www.youtube.com/watch?v=zp7NtW_hKJI)
0 commit comments